2: Creating a static website
Canister smart contracts are able to serve HTTP content natively, allowing for dapp frontends to be served directly in a web browser using the canister's URL at http://<canister id>.icp0.io
and http://<canister id>.raw.icp0.io
. Frontend canisters can be used to deliver HTML, CSS, and JavaScript pages and answer API requests.
If a canister wants to serve HTTP content, it should implement a method that consumes an HTTP request, which contains a URL, HTTP method, and headers, then outputs an HTTP response that contains a status, headers, and the response body. The canister method can return HTML, CSS, and JavaScript content as part of the HTTP response.
To compile frontend assets like CSS and JavaScript into a Wasm module that can be deployed to ICP as a canister, dfx
uses Rust boilerplate code to create an asset canister.
The terms 'asset canister' and 'frontend canister' are sometimes used interchangeably. Asset canister is typically used to describe the Rust code dfx
uses in the background to compile a project's frontend assets into Wasm. Frontend canister is typically used as a general term to describe a project's frontend.
Applications deployed on ICP can have frontends that:
Are deployed on ICP and communicate with backend canister(s) to create a fully onchain dapp.
Are hosted externally (off-chain) and communicate with backend canister(s) deployed on ICP.
Only provide web assets and do not communicate with a backend canister.
In the previous tutorial, you deployed a project with a single backend canister and then interacted with that canister through the Candid API interface. In this tutorial, let's explore how a canister can host a static website that does not interact with a backend canister and is used only to serve web assets.
Crypto blog example
Open the ICP Ninja 'My Crypto Blog' project. View the ICP Ninja demo video for more details about using the platform.
Let's review the project's file structure:
├── frontend # Folder containing the asset files for your dapp's frontend.
│ ├── public
| │ ├── favicon.ico
│ ├── src
| │ ├── main.jsx # Source code for a simple React application.
│ ├── index.css
│ ├── index.html
│ ├── package.json
│ ├── postcss.config.js
│ ├── tailwind.config.js
│ ├── vite.config.js
├── dfx.json # The configuration file for your Internet Computer dapp.
├── package-lock.json
├── package.json
├── README.md # Information about the project and using ICP Ninja.
dfx.json
The dfx.json
file for this project will closely resemble the one used for the Hello, world! example; however there is one key difference. Rather than defining what the canister is "type": "motoko"
, this project will define the canister as "type": "asset"
which prompts dfx
to compile the canister's assets into a Wasm module using Rust boilerplate code.
{
"canisters": {
"frontend": {
"frontend": {
"entrypoint": "frontend/index.html"
},
"source": ["frontend/dist"],
"type": "assets"
}
}
}
frontend/src/main.jsx
This project's main.jsx
file defines the source code for a simple app using React, Vite, and Tailwind CSS that the canister will host. This app will create a very basic blog website with three randomly generated placeholder blog post entries.
import React, { useState } from 'react';
import ReactDOM from 'react-dom/client';
import '../index.css';
function App() {
const [data, setData] = useState(null);
const [isLoading, setIsLoading] = useState(false);
const [error, setError] = useState(null);
const fetchData = async () => {
setIsLoading(true);
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error('Network response was not ok');
}
const result = await response.json();
setData(result);
} catch (err) {
setError(err.message);
} finally {
setIsLoading(false);
}
};
return (
<div className="flex min-h-screen items-center justify-center bg-gradient-to-r from-blue-400 to-purple-500">
<div className="w-96 rounded-lg bg-white p-8 shadow-lg">
<h1 className="mb-4 text-center text-3xl font-bold text-gray-800">🚀 My Crypto Blog 🚀</h1>
<p className="text-center text-gray-600">
A simple web page hosted onchain on ICP. Built with React, Vite, and Tailwind CSS.
</p>
<br></br>
<p className="text-center text-gray-600">
You can host any kind of frontend application, including React, Vue, Svelte, and more on ICP!
</p>
<div className="mt-6 flex justify-center">
<button onClick={fetchData} className="rounded bg-blue-600 px-4 py-2 font-bold text-white hover:bg-blue-700">
Fetch Posts
</button>
</div>
{isLoading && <p className="mt-4 text-center text-gray-600">Loading...</p>}
{error && <p className="mt-4 text-center text-red-500">Error: {error}</p>}
{data && (
<div className="mt-4">
{data.slice(0, 3).map(
(
post // Display only first 3 posts for brevity
) => (
<div key={post.id} className="mb-2 rounded bg-gray-100 p-2">
<h3 className="text-lg font-semibold">{post.title}</h3>
<p className="text-sm">{post.body.slice(0, 100)}...</p>
</div>
)
)}
</div>
)}
</div>
</div>
);
}
export default App;
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
If you want to learn more about this code, you can right-click and select "Ask AI - Explain" to get a more detailed breakdown of the code's syntax and components.
Deploying the canister
Click the "Deploy" button in the upper right corner of the code editor. ICP Ninja will deploy the project and return the canister's URL:
→ Reserving canisters onchain
→ Building frontend
→ Uploading frontend assets
🥷🚀🎉 Your dapp's Internet Computer URL is ready:
https://rueiq-3qaaa-aaaab-qbk7q-cai.icp1.io
⏰ Your dapp will be available for 20 minutes
Open the dapp's "Internet Computer URL" in your web browser. This will open the React application, which will include a button you can click to generate the three random blog posts.
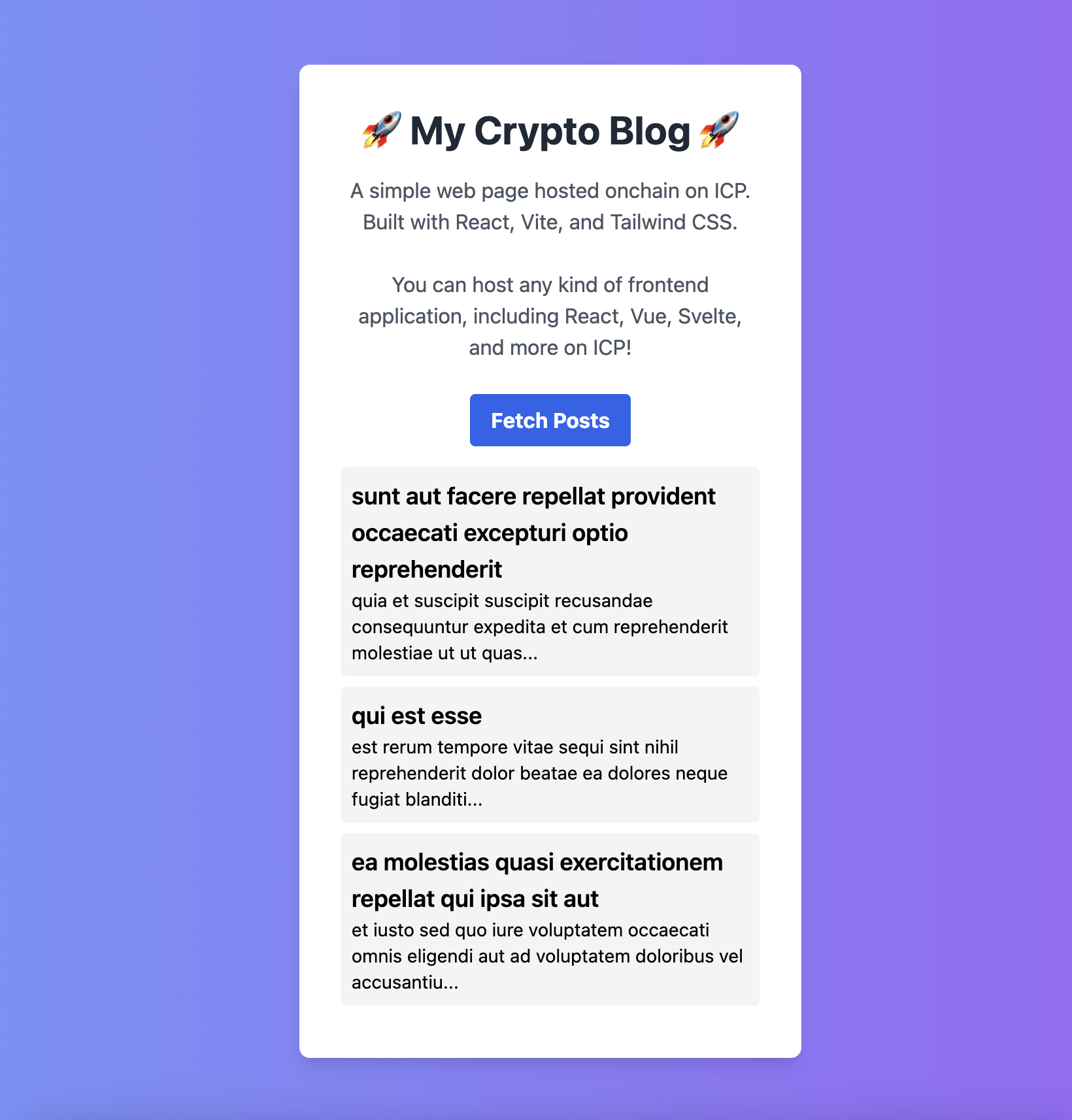
To edit the website's content, make adjustments to either the main.jsx
file or change the styling in the index.css
file. For example, you can change the page's title, body text, or button functionality, or add photos and videos to be embedded on the page.
Since this is a static website, however, if you'd like to add any backend functionality such as user authentication and profiles, saving data to a user's account, or uploading and editing data, you will need to add a backend canister to your project.